PNG-Bilder mit Java
Als ich vor der Frage stand, mit welcher Sprache man einfach Bilder zeichnen und in ein File schreiben kann, habe ich wieder mal die Java-Library genauer angesehen.
Ueberraschung: es braucht bloss eine einzige Zeile Code um ein Bild in ein File zu schreiben! Dummerweise kann diese Zeile eine Exception werfen, so dass ein paar zusätzliche Zeilen nötig sind um die Exception zu behandeln.
Dieses Beispiel benutzt AWT-Funktionen um das Bild zu zeichnen, aber BufferedImage bietet auch ein 2D Graphics an das mehr und komfortablere Methoden hat.
Der Code
Bis auf die Bitoperationen in der draw-Methode und den Trick mit der Symmetrie ist der Code eigentlich sehr einfach zu durchschauen:
/******************************************************************************/ /* */ /* FILE: CreatePNG.java */ /* */ /* Draws an image and writes it to a file */ /* ====================================== */ /* */ /* V1.00 25-JUL-2015 Te https://www.heimetli.ch */ /* */ /******************************************************************************/ import java.awt.* ; import java.awt.image.* ; import java.io.* ; import javax.imageio.* ; /** * Draws an image and writes it to a .PNG file */ public class CreatePNG { static final int SIZE = 295 ; BufferedImage img ; /** * Creates the bitmap and fills the background * @param size Size of the bitmap in pixels (the bitmap is quadratic) */ public CreatePNG( int size ) { img = new BufferedImage( size, size, BufferedImage.TYPE_INT_RGB ) ; Graphics g = img.getGraphics() ; g.setColor( Color.white ) ; g.fillRect( 0, 0, SIZE, SIZE ) ; } /** * Draws an element onto the bitmap. * The coordinate system is unusual to simplify the draw method. * @param g Graphics of the image * @param x X-Coordinate of the element * @param y Y-Coordinate of the element */ void drawElement( Graphics g, int x, int y ) { g.fillRect( 4 + (8 + x) * 17, 4 + (8 + y) * 17, 15, 15 ) ; } /** * Draws the image in the byte array. * Because the image is symmetric, one definition is sufficient to * draw all four parts of the picture. * @param def Definition for the elements */ private void draw( byte def[] ) { Graphics g = img.getGraphics() ; g.setColor( Color.blue ) ; for( int y = 0; y < def.length; y++ ) { for( int x = 0; x < 8; x++ ) { if( (def[y] & (1 << x)) != 0 ) { drawElement( g, x, y ) ; drawElement( g, x, -y ) ; drawElement( g, -x, y ) ; drawElement( g, -x, -y ) ; } } } } /** * Writes the image to the given file * @param filename Name and path for the file */ private void write( String filename ) { try { ImageIO.write( img, "png", new File(filename) ) ; } catch( IOException e ) { e.printStackTrace() ; } } /** * Main program * @param args Unused */ public static void main( String args[] ) { CreatePNG cp = new CreatePNG( SIZE ) ; cp.draw( new byte[] { (byte)0xDE, 0x0D, 0x1B, 0x07, 0x05, 0x00, 0x01, 0x01 } ) ; cp.write( "java.png" ) ; } }
Das erzeugte Bild
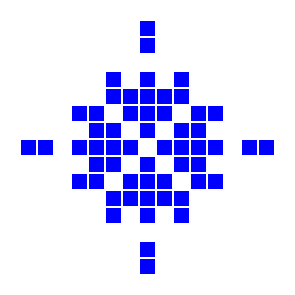
Der Source-Code
Sie können CreatePNG.java herunterladen und selber damit spielen.
Aus den Bildern ein animiertes GIF erzeugen
Wenn Sie bis hierher gelesen haben, dann könnten Sie auch daran interessiert sein, aus mehreren Bildern ein animiertes GIF zu machen.