Die Langton-Ameise
Christopher Langton ist ein amerikanischer Computerwissenschaftler der sich mit "Artificial Life" beschäftigte.
Dabei erfand er einen einfachen Algorithmus der eine Ameise simulieren sollte. Die Ameise läuft in einem Gitter von Feld zu Feld, und hinterlässt dabei eine Spur. Je nachdem welche Farbe das aktuelle Feld hat dreht sie nach rechts oder links. Eine genauere Beschreibung finden Sie in der Wikipedia.
Die folgende Visualisierung stellt eine solche Spur dar:
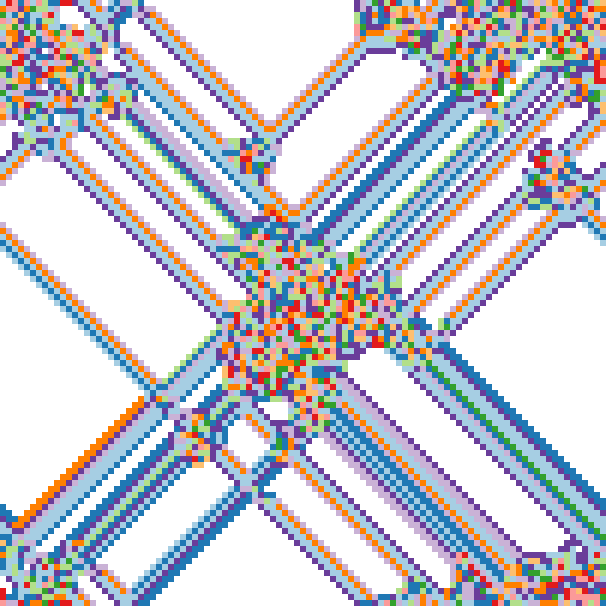
Bemerkenswert ist dabei dass die chaotische Darstellung nicht durch Zufall entsteht, sondern deterministisch ist. Bei jedem Lauf entsteht wieder das genau gleiche Bild.
Die Ameise in Aktion
Der Code dazu
class Ant { static colors = ["#a6cee3","#1f78b4","#b2df8a","#33a02c","#fb9a99","#e31a1c","#fdbf6f","#ff7f00","#cab2d6","#6a3d9a"] ; static SIZE = 101 ; constructor() { this.ctx = document.querySelector( "canvas" ).getContext( "2d" ) ; this.x = 50 ; this.y = 50 ; this.direction = 0 ; this.steps = [ this.up, this.right, this.down, this.left ] ; let template = new Array( Ant.SIZE ).fill( 0 ) ; this.fields = new Array( Ant.SIZE ) ; for( let i = 0; i < Ant.SIZE; i++ ) this.fields[i] = template.slice() ; setInterval( this.step, 10, this ) ; } up( self ) { if( --self.y < 0 ) self.y = Ant.SIZE - 1 ; } right( self ) { if( ++self.x >= Ant.SIZE ) self.x = 0 ; } down( self ) { if( ++self.y >= Ant.SIZE ) self.y = 0 ; } left( self ) { if( --self.x < 0 ) self.x = Ant.SIZE - 1 ; } step( self ) { let value = self.fields[self.x][self.y] + 1 ; if( value == Ant.colors.length ) { self.direction = (self.direction + 1) & 3 ; value = 0 ; } else self.direction = (self.direction - 1) & 3 ; self.fields[self.x][self.y] = value ; self.paintField() ; self.steps[self.direction]( self ) ; } paintField() { this.ctx.fillStyle = Ant.colors[this.fields[this.x][this.y]] ; this.ctx.fillRect( this.x * 6, this.y * 6, 6, 6 ) ; } } const ant = new Ant() ;